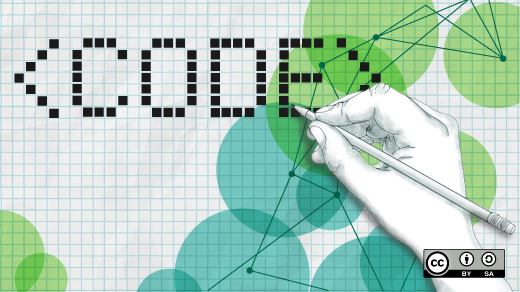
Exploring the history of technology
The standard recommendation for how to get started in open source is to “scratch an itch,” but with so many great open source programs and applications already available for every platform, including the web, it might be difficult to find your starting point. I’d like to make a new suggestion to anyone who wants to get started in open source programming: explore technology history by writing a few cool “retro” programs.
Here are a few “jumping off” points that any new programmer can use to learn both about programming and about the history of computing. Try out these ideas to explore a programming language that’s new to you, whether that’s Python, Rust, Go, or any other programming language.
fmt: The trivial document formatter
When early Unix started to become popular as a research platform, universities wanted to learn more about it. The University of California at Berkeley requested and received a copy of Unix to run on a computer system there. Over time, faculty and students at Berkeley updated this copy of Unix and added new features to it. This “Berkeley Unix” became “BSD,” the Berkeley Software Distribution; BSD remains a popular version of Unix.
One useful utility added to BSD was fmt
, a program that reformatted plain text files. In its most basic usage, fmt
collected words from the input and filled paragraphs on the output. The modern fmt
program accepts several command line arguments that control formatting, such as -c
to center the output lines and -m
to recognize mail headers. However, a simple implementation of fmt
might only accept files as command line arguments, and make assumptions for how the program should operate, such as hard-coding an output width.
This program is a good exercise to learn a new programming language, and should only require functions to read lines of data and recognize words or tokens in the input. One implementation might print words one at a time to the output. Keep track of the length of the “current output line, and the length of the next word to print, and add a new line when the line gets too long.
RUNOFF: An early document markup system
RUNOFF was certainly not the first document preparation system, it borrowed ideas from several predecessors that came before it. But RUNOFF made it easy to format documents for later printing on a chain printer. In the 1960s, these typewriter-like printers were very common.
Like fmt
, RUNOFF collected words from a file then filled paragraphs for the output. Blank lines started a new paragraph. This was the most basic way to format documents.
But the real power of RUNOFF was using its control words to format text. These commands started with a leading period in the first column of a line, such as .center
to center the next line of text, which could be abbreviated as .ce
as a two-letter command.
To write your own version of RUNOFF, start with an implementation of fmt
that reads input from a file, and fills one line of text at a time. Then add control words to provide basic formatting. A simple implementation of RUNOFF might only use the abbreviated control words, like .br
to “break” a new line, and .bp
to begin a new page.
Control word | Abbreviation | Causes break? |
.adjust | .ad | yes |
.append FILE | .ap FILE | no |
.begin page | .bp | yes |
.break | .br | yes |
.center | .ce | yes |
.double space | .ds | yes |
.fill | .fi | yes |
.header TEXT | .he TEXT | no |
.indent N | .in N | no |
.line length N | .ll N | no |
.literal | .li | no |
.nofill | .nf | yes |
.nojust | .nj | yes |
.page (N) | .pa (N) | yes (if N) |
.single space | .ss | yes |
.space (N) | .sp (N) | yes (default: N=1 line) |
RUNOFF is an excellent program to learn a new programming language. And it demonstrates the usefulness of other document processing systems. In fact, programmers at Bell Labs found RUNOFF so useful that they created their own implementation for the first Unix system. This early version only implemented the abbreviated commands, and so it was given an abbreviated name: roff
. Later, the Unix team added new features to roff
to support more advanced document formatting; this “new roff” was called nroff
. And several years later, when Bell Labs acquired a C/A/T phototypesetter, they updated nroff
to support fonts and other special formatting provided by the C/A/T; this “typesetter roff” was named troff
.
LANPAR: The first computer spreadsheet program
In the 1960s, computers were still new to organizations. But computers of that era were not yet like the computers we know today: instead of interacting with the system with a keyboard and mouse, and seeing your results on a screen, computer operators fed stacks of cards into the system and waited until the computer processed them and printed the output on paper. It was a slow process compared to day’s immediate user feedback, but these computers were a boon to productivity in the 1960s.
For example, managers of business departments would need to create annual budget plans. These were models of what the organization expected to do over the next year, including spend and revenue. Modeling budgets was very tedious to do on paper, so the central computing departments in most organizations developed finance planning programs. Enter your starting assumptions and the finance software would plan your spend, estimate your revenue, and calculate your budget. But if you wanted to model a different set of expectations, it might take the programming team several months to implement even a minor change.
In 1969, two engineers at Bell Canada had a pretty neat idea: “What if managers could describe their own budget assumptions and calculations in a way that made sense to them? The system can model a new budget with that data and programmers can focus on more interesting problems.” The result was a new program called LANPAR that was the first computer spreadsheet program, although not in the form we might recognize today.
Here’s how LANPAR worked: Imagine a grid of cells or “boxes” that each contain either a number or a calculation. In LANPAR, everything was a calculation or a number, no text. To define a number, use the letter K followed by the value, like this:
K 3.141
Calculations used references to other boxes in the grid. The first row of boxes was 101, 102, 103, and so on. The second row was 201, 202, 203, … so any box address was two digits for the “column” and one or more digits for the “row.” LANPAR supported 99 columns and 999 rows.
101 | 102 | 103 | 104 |
201 | 202 | 203 | 204 |
301 | 302 | 303 | 304 |
A calculation used these box references with basic arithmetic operations, in the order presented. LANPAR did not use operator precedence, so adding a few numbers then dividing the sum by another number looked like this:
101 + 102 + 103 / 201
For example, if the first row of numbers was 1, 2, and 3, and box 201 had the number 3, the calculation would be the same as 1+2+3=6, then 6 divided by 3, giving the value 2.
Originating in 1969, LANPAR could only read data from punched cards, rather than keyboard input. Each card represented a row of boxes; punched cards were limited to 72 columns of data, so LANPAR also supported continuation cards. But to explore this retro-computing program, your modern version of LANPAR might read lines of data from a file. Separate box data with a semicolon, like the original LANPAR. For example, to enter a simple calculation to find the average of three numbers, you might enter these lines: One line to hold the data, one box on a new line with the count, and another box on a third line to perform the calculation.
K 1 ; K 2 ; K 3
K 3
101 + 102 + 103 / 201
Because LANPAR operated in “batch” mode, it read the data, then processed all the boxes in a loop. When all calculations were complete, it printed the results. So the above LANPAR “spreadsheet” file might generate output like this:
1 ; 2 ; 3
3
2
This is a great first program to explore a new programming language. Writing your own version of LANPAR requires arrays to hold the data, and loops to iterate through the boxes to perform the calculations. Any LANPAR implementation will need to convert string data into number values, such as K 3
to become the number 3. This will also require a parser for the data to read each part of the data.
Learn programming and explore tech history
Whether you’re new to programming or learning a new programming language, these project ideas can be great “jumping off” points to writing a new program. And I love that these programs demonstrate some key milestones in computing history: For example, LANPAR was the first computer “spreadsheet” and inspired other desktop spreadsheet programs like VisiCalc and Lotus 1-2-3. RUNOFF wasn’t the first computer document preparation system, but it influenced other systems that came after it.
So by exploring these programs and writing your own versions, you can learn a new programming language and also gain first-hand knowledge about how these programs worked. This is an excellent exercise for any programmer, at any skill level.